前言
使用 wkhtmltopdf 实现转换 PDF 时,是否可以设置自定义页眉和页脚内容?wkhtmltopdf 作为一个命令行工具,它提供了全局参数、大纲参数选项、页面对象参数、页眉和页脚参数选项和目录对象参数五种命令参数。本文介绍页眉和页脚参数选项实现自定义页眉和页脚内容。
命令参数
1、页眉和页脚参数
--header-center [text]:在页眉中居中指定文本;
--header-left [text]:将文本放置在页眉的左侧;
--header-right [text]:将文本放置在页眉的右侧;
--header-html [url]:允许对标题使用自定义的 HTML 文件【包含格式化文本、图像等】;
--footer-center [text]:在页脚中居中指定文本;
--footer-left [text]:将文本放在页脚的左侧;
--footer-right [text]:将文本放置在页脚的右侧;
--footer-html [url]:允许对页脚使用自定义的 HTML 文件【包含格式化文本、图像等】;
页眉或页脚参数的text用下面元素替换,则可显示对应的内容。如 --footer-center "[page] of [topage]"
[page]:当前页码
[toPage]:总页数
[date]:当前日期
[time]:当前时间
[title]:文档标题
[subTitle]:文档副标题
[pageNumber]:页码
[totalPages]:总页数
#region 页眉
--header-spacing [value]:控制页眉与内容之间的间距;
--header-font-size [size]:设置标题文本的字体大小;
--header-line:在页眉下方显示一条直线分隔正文;
#endregion
#region 页脚
--footer-spacing [value]:控制页脚与内容之间的间距;
--footer-font-size [size]:设置页脚文本的字体大小;
--footer-line:在页脚上方显示一条直线分隔正文;
#endregion
2、页面对象参数
#region 部分
--print-media-type:用显示媒体类型代替屏幕;
--no-print-media-type:不用显示媒体类型代替屏幕;
--page-offset <offset>:设置页码的起始值(默认值为0);
--encoding <encoding>:为输入的文本设置默认的编码方式;
--zoom <float>:设置转换成PDF时页面的缩放比例(默认为1);
#endregion
3、全局参数
#region 部分
--margin-bottom <unitreal> 设置页面的 底边距;
--margin-left <unitreal> 设置页面的 左边距 (默认是 10mm);
--margin-right <unitreal> 设置页面的 右边距 (默认是 10mm);
--margin-top <unitreal> 设置页面的 上边距;
--page-height <unitreal> 页面高度;
--page-size <Size> 设置页面的尺寸,如:A4,Letter等,默认是:A4;
--page-width <unitreal> 页面宽度;
--quiet 静态模式,不在标准输出中打印任何信息;
#endregion
4、命令参数详解附录
https://wkhtmltopdf.org/usage/wkhtmltopdf.txt
自定义示例
1、转换的HTML文件
<!DOCTYPE HTML>
<html>
<head>
<meta charset="gbk">
<title>测式文件</title>
</head>
<body>
<div id="sse">
<input id="url" size=200 value="ws://127.0.0.1:8080/service" /><button id="btn1" onclick="changewebsocket(this)" tt=1>打开连接</button><br>
<input id="msg" size=200 value='测试内容'/>
<button onclick="sendmsg()">发送数据</button><br>
<textarea id="onmsg" rows="10" cols="30"></textarea>
</div>
</body>
</html>
2、作为页眉的HTML 文件
<!DOCTYPE html>
<html lang="en">
<head>
<title>
Testing
</title>
</head>
<body>
<table cellpadding="0" cellspacing="0" border="0" style="width:100%">
<tr>
<td style="max-width:40%">
<img alt="text" src="https://profile-avatar.csdnimg.cn/7d678480185a4ae5babed86c378e532e_funniyuan.jpg!1"
style="max-width:100%">
</td>
<td style="max-width:60%">
<table cellpadding="0" cellspacing="0" border="0" style="width:100%">
<tr>
<td align="center" style="font-size:30px;color:#e14a3a;font-family:SimHei;font-weight:600;padding:15px 0 5px">
Company Name
</td>
</tr>
<tr>
<td align="center" style="font-size:16px;color:#0a0f84;font-family:SimHei;padding-bottom:10px">
Invoice
</td>
</tr>
</table>
</td>
</tr>
<tr>
<td colspan="2" style="width:100%;border-width:1px;border-style:solid;border-color:#000">
</td>
</tr>
</table>
</body>
</html>
3、实现与调用
using System.Diagnostics;
namespace Fountain.WinConsole.ToPDFOrImageDemo
{
public class ConverterPDF:IConverterEngine
{
/// <summary>
/// wkhtmltopdf 工具路径
/// </summary>
public string ConverterPath { get; }
/// <summary>
/// 转换类型
/// </summary>
public int EngineType { get; } = 1;
/// <summary>
///
/// </summary>
/// <param name="converterPath"></param>
public ConverterPDF(string converterPath)
{
ConverterPath = converterPath;
}
/// <summary>
///
/// </summary>
/// <param name="htmlPath"></param>
/// <param name="outputPath"></param>
/// <returns></returns>
public bool Convert(string htmlPath, string outputPath)
{
try
{
var ticks = DateTime.UtcNow.Ticks;
string optionSwitches = "";
#region 页眉
// 设置标题字体大小
optionSwitches += "--header-font-size 10 ";
// 将 header.html 作为页眉内容
optionSwitches += "--header-html header.html ";
#endregion
#region 页面
// 使用的打印介质类型,而不是屏幕
optionSwitches += "--print-media-type ";
// 边距
optionSwitches += "--margin-top 40mm --margin-bottom 10mm --margin-right 10mm --margin-left 10mm ";
// 纸张大小
optionSwitches += "--page-size A4 ";
#endregion
#region 页脚
//
optionSwitches += "--footer-font-size 8 ";
// 在页脚的居中部分显示页脚文本
optionSwitches += "--footer-right \"[page]/[topage]\" ";
#endregion
Process process = new Process();
process.StartInfo.UseShellExecute = true;
process.StartInfo.FileName = this.ConverterPath;
process.StartInfo.Arguments = $"{optionSwitches} \"{htmlPath}\" \"{outputPath}\" ";
process.Start();
}
catch (Exception ex)
{
throw new Exception("转PDF出错", ex);
}
return true;
}
}
}
using System.Text;
namespace Fountain.WinConsole.ToPDFOrImageDemo
{
internal class Program
{
static void Main(string[] args)
{
var ticks = DateTime.UtcNow.Ticks;
string outputpdf = $"{AppDomain.CurrentDomain.BaseDirectory}{ticks}.pdf";
string htmlPath = $"{AppDomain.CurrentDomain.BaseDirectory}test.html";
string convertPath= $"{AppDomain.CurrentDomain.BaseDirectory}wkhtmltopdf.exe";
ConverterPDF converter = new ConverterPDF(convertPath);
converter?.Convert(htmlPath, outputpdf);
Console.ReadKey();
}
}
}
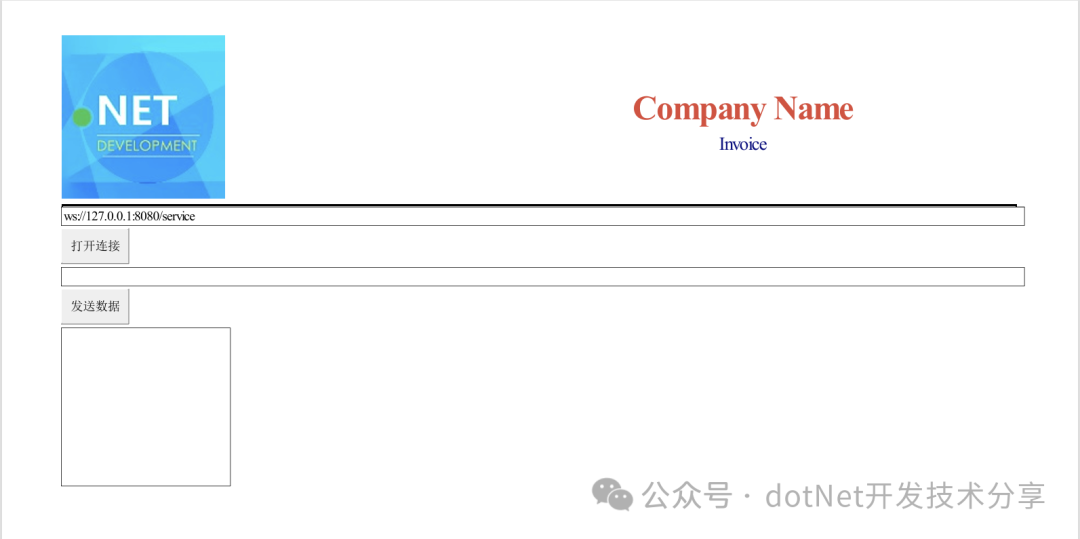
小结
以上是页眉和页脚参数选项内容介绍,并通过以个示例,了解其实现自定义页眉和页脚内容的方式。
该文章在 2024/11/18 9:05:00 编辑过